Khtml Maplib API
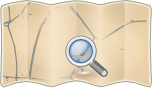
- About
- This page describes a JavaScript API for slippy maps.
- Reason for being historic
- This project is dead. Its author recommends to use Leaflet instead.
- Slippy map for WebBrowsers
- WGS84
- OSM and other tiles
- Markers Module
- Vectorgraphics Module
- Overlay API
Audience
Javascript Programmers
Description
This was a Public Domain documentation for a map API used in Web Browsers. There are NO librarys that implemented this API at the moment. The khtml.maplib was supposed to implement this API, but has been discontinued, and KHTML itself has also been discontinued by KDE.
The documentation describes a very simple but complete API for Browser Maps. It should be possible to use this api for benchmark tests.
- The target is to have an easy to use map library for html coders and end user.
- The API should be stable and should not be changed in future.
- The API should be complete and provide functionality for higher level functions (kml,...).
- The API should be competition inviting.
- This API describe and absolute minimum of functionality. This is like DOM, Jquery,... can use it.
- It should be possible to make benchmark tests which is measured by frames per second.
- The library should provide a full mouse and|or touchscreen ui.
- The map should be fast and resource-efficient
- The lib must be xss save.
Syntax
Methods
Setter and getter have the same method name, example:
//set center of map to a new position
map.center(center);
//get center of map coordinates
center=map.center();
If you call a method the map will render the changes you make automaticaly.
Base API
//choose map render libary
var mr=new Object(khtml.maplib);
Version option would be nice
LatLng
- constructor: lat, lng
- methods: lat, lng
example
//constructor
var p=new mr.LatLng(48.2,15.6);
//set lat/lng of existing point
p.lat(48.21);
p.lng(15.65);
//read lat/lng
var lat=p.lat();
var lng=p.lng();
The LatLng Object is not visible. The LatLng Object handles all coordinates of all visible or invisible thing.
Bounds
- constructor: point1, point2
- methods: sw, ne, getDistance (returns the distance between the points in meter)
example
//the globe fits to the div
var map=new mr.Map(document.getElementById("map"));
var p1=new mr.LatLng(-70,-180);
var p2=new mr.LatLng(70,180);
var b=new mr.Bounds(p1,p2);
map.bounds(b);
alert("do you see the children all over the world?")
//get southwest coordinate
var sw=b.sw();
alert(sw.lat());
//set a new coordinate to existing bounds
var p3=new mr.LatLng(34.5799,23.83);
b.sw(p3);
map.bounds(b); //content of map view is changed
//get Distance in meter
var d=b.getDistance();
alert(d+" meter");
getDistance does not really fit to the bounds. This function should maybe go to the LatLng object like: var distance=p1.distance(p2); alert(distance+" Meter");
Map
- constructor: domElement - mounts the map to a div
Position and Zoomlevel
- centerAndZoom:
mr.LatLng Number Return null (nothing)
- center:
mr.LatLng return mr.LatLng
- zoom: zoomlevel
return number
- bounds:
mr.LatLng mr.LatLng return mr.Bounds
- moveXY: deltaX,deltaY (pixel)
Overlay Handling
- addOverlay: vectors, markers, ui component
- removeOverlay: overlay
simple callback function
- addCallbackFunction: user defined function called if the map is moved or zoomed
- removeCallbackFunction
workaround for slow browsers (offsetWidth,..)
- redraw: has to be called if the div changes position or size is changing (offsetWidth, offsetHeigt are very slow in 2010 browsers)
Animation
- animatedGoto(new khtml.maplib.LatLng(lat,lng),zoom,time); //time in milliseconds
for internal use
- latlngToPixel
- PixelTolatlng
- map.size.left; // map.size.left => left gap to browser window in pixel
- map.size.top;
- map.size.width;
- map.size.height;
- mouseToLatLng(event)
example
//initialize the map
var map=new mr.Map(document.getElementById("map"));
var p=new mr.LatLng(48.2,15.6);
map.centerAndZoom(p,12);
What's the difference between callbackfunction and overlay? Could the callbackfunction be an overlay to keep the core simple? removeAllOverlays is a stupid method. It would be more interesting to get a list of the layers.
Overlay API
There is an extra page for Geometry Overlays: [1]
Marker
There is an extra page for Simple_marker_API.
mr.LatLng domElement options {dx:10,dy:10,minimum-zoom:3,maximum-zoom:12}
example
var img=document.createElement("img"); //DOM 2.0
img.setAttribute("src","markerimage.png"); //DOM 2.0
var p=new mr.LatLng(48.2,15.6);
var marker=new mr.Marker(p,img,{dx:"15px",dy:"32px"}); //it is possible to add DOM Objects that represents html, svg,...
map.addOverlay(marker);
//move a marker
marker.geometry.coordinates.lat(28);
marker.render();
What if the Point p will get coordinates using p.lat(48.22); Will the marker move?
margin-top works only for positiv values and negativ values > -10
There should be an option for min/max zoomlevel
How to move a marker 50 meter to the east
Overlay for Vector Data and Markers
Handling visible Overlays is not part of the base API.
- There is an extra page for | Vector Overlays
- There is an extra page for | Markers
Changing tile source
map.tiles({
maxzoom:18,
minzoom:1,
src:function(x,y,z){
return "http://andy.sandbox.cloudmade.com/tiles/cycle/"+z+"/"+x+"/"+y+".png";
},
copyright:"osm"
})
Tile overlays (PNG with opacity)
ov=map.addTilesOverlay({
maxzoom:18,
minzoom:4,
src:function(x,y,z){
return "http://tiles.openseamap.org/seamark/"+z+"/"+x+"/"+y+".png";
},
copyright:"openseamap"
})
//remove tile overlay
map.removeTilesOverlay(ov);
missing
- splines,...
Full example in Prefix Notation
<!DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 4.01//EN" "http://www.w3.org/TR/html4/strict.dtd">
<html>
<head>
<style type="text/css">
#map{
width:400px;
height:300px;
}
</style>
<script type="text/javascript" src="path/to/maplib.js">
<script type="text/javascript">
var mr=new Object(khtml.maplib);
var map=null; //global map object
function init(){
map=new mr.Map(document.getElementById("map"));
var center=new mr.LatLng(48.2,15.6);
map.centerAndZoom(center,12); //12 = zoomlevel
//now the map is working, if you don't need overlays you are finished
//marker at center
var img=document.createElement("img");
img.setAttribute("src","marker.png");
var marker=new mr.Marker(center,img);
map.featureCollection.appendChild(marker);
//add polyline
var pArray=new Array();
pArray.push(new mr.LatLng(48.2,15.6);
pArray.push(new mr.LatLng(48.21,15.8);
pArray.push(new mr.LatLng(48.22,15.3);
pArray.push(new mr.LatLng(48.24,15.2);
var line=new mr.geometry.Feature({type:"LineString",coordinates:pArray);
line.style.stroke="blue";
line.style.fill="red";
map.featureCollection.appendChil(line);
}
</script>
</head>
<body onload="init()">
<div id="map"> </div>
</body>
</html>
User Interface
Mouse-, Touch-, Multitouch- events are handled by the lib. Visible UI Parts are easy to implement and part of the extensions.
Keyboard Support
The map needs the focus for handling key events. The map must not eat <crtl>-r of tab event. Firefox needs tabindex to set focus on a div.
key | keycode | action |
---|---|---|
plus | 187;61 | zoom in |
minus | 189;109 | zoom out |
cursor left | 37 | short press moves 10px, long press starts animation |
cursor right | 39 | |
cursor down | 40 | |
cursor up | 38 | |
home | 36 | map moves left 80%, ease in, ease out animation |
end | 35 | |
page up | 33 | |
page down | 34 | |
<shift> + mouse | draw rect for zoom | |
<ctrl> +mouse | distance measure tool |
Extensions
The Vector Class renders the vector data on diffent backends. Very common are SVG, VML and Canvas. It calculates all the WGS84 to pixel coordinate. Markers are placing domElements on the map. Instead of doing on css:top/left you use WGS84 Coordinates. The Vector and Marker Interface should be highly optimized for speed. Optimization for speed is the main goal of the lib and higher level functions provied from this speed. Extension Programmers have a reliable easy to use API.
For different coordinate system the point Class has to be extended. Extensions should use the Vector and Marker Class.
Possible Extentions
Vector
- kml
var xmlhttp=new XMLHttpRequest();
xmlhttp.open("GET","test1.kml",true);
xmlhtp.onreadystatechange=function(){
if(xmlhttp.readyState==4){
render(xmlhttp.responeseXML);
}
}
function render(kml){
var renderer=new Kml(kml);
map.addOverlay(renderer);
}
- osm
Rendering OSM XML an Vector Graphics is similar to kml rendering. osm redenering additionaly need a style definition which could be borrowed from osma
- gml
- gpx
Same way as kml, but much easier.
What do you need from base Layer?
Visible User Interface
- Zoom slider - be fancy and use webGL
- show lat/lon/zoom
Use addCallbackFunction for UI updates
function displayCoordinates(themap){
var center=themap.center();
document.getElementById("coords").textContent=lat:"+center.lat()"+,"lng: "+center.lng();
}
map.addCallbackFunction(displayCoordinates);
...
<div id="coords"> </div>
Animations
map.goto(point,zoom,duration in sec);
example
map.goto(new point(48.2,15.6),12,2);
Keyboard
There are some problems with focus finding if there are many maps on the same html page. Even to get the keyboard focus to the map is not an easy task.
Integration
- DOM
The map is mounted to a DOM Element. The maplib must read the size and position of this DIV. The DIV must be fully styleable by CSS. Also the padding parameter must work same an with img-elements.
- jquery
$(".map").makeMap(lat,lng,zoom);
//add the map to all div with class "map"
$(".map").makeMap(48.2,16.54,14);
- wordpress
Programming Overlays
function Spline(...params...){
//... your code ...
this.init=function(themap){
//... your code for intializing...
}
this.render(){
//... is called if map is moved or zoomed ...
}
this.clear(){
//... for canvas renderer, to clear the drawing...it's called only once per frame.
}
this.destroy(){
//...remove all visible parts from map. Delete Variables.
}
}
var splines=new Spline(...params..);
map.addOverlay(splines);
Discussion
There should be a prefix like in Google maps api v3 for all classes.
Units
Numbers without units are WGS84 Coordinates. It should be possible to do nearly everything using "px" or "m" Units.
The moveXY or the circle radius could take parameters in m, px, und wgs84 .
Other methods maybe can't handle all kind of parameters.
Error Handling
How to handle that?